Mastering Front-End Development: A Comprehensive Guide from Basics to Advanced Techniques
I still remember the day I wrote my first line of HTML code - it was messy, probably had more closing tags than opening ones, and looked nothing like the sleek websites I admired. But that single step launched me into the fascinating world of front-end development, a journey that's been equal parts frustrating and rewarding. Front-end development isn't just about making things look pretty (though that's definitely a perk) - it's where art meets functionality, where your code transforms into something people can see, touch, and interact with. It's like being given a blank canvas, some paints, and being told "make something beautiful that also works perfectly." No pressure, right? From those first tentative steps with HTML to wrestling with CSS to make things align (spoiler alert: they never align the first time) to the magical moment when your JavaScript actually does what you intended, the path of a front-end developer is one of constant learning, occasional head-banging on keyboards, and those glorious "aha!" moments that make it all worthwhile. In this article, I want to take you on that journey - not as some expert who never makes mistakes, but as someone who's been there, broken things, fixed them, and learned along the way. Whether you're just starting out or looking to add some new skills to your developer toolbelt, I hope my experiences and insights can make your path a little smoother than mine was.
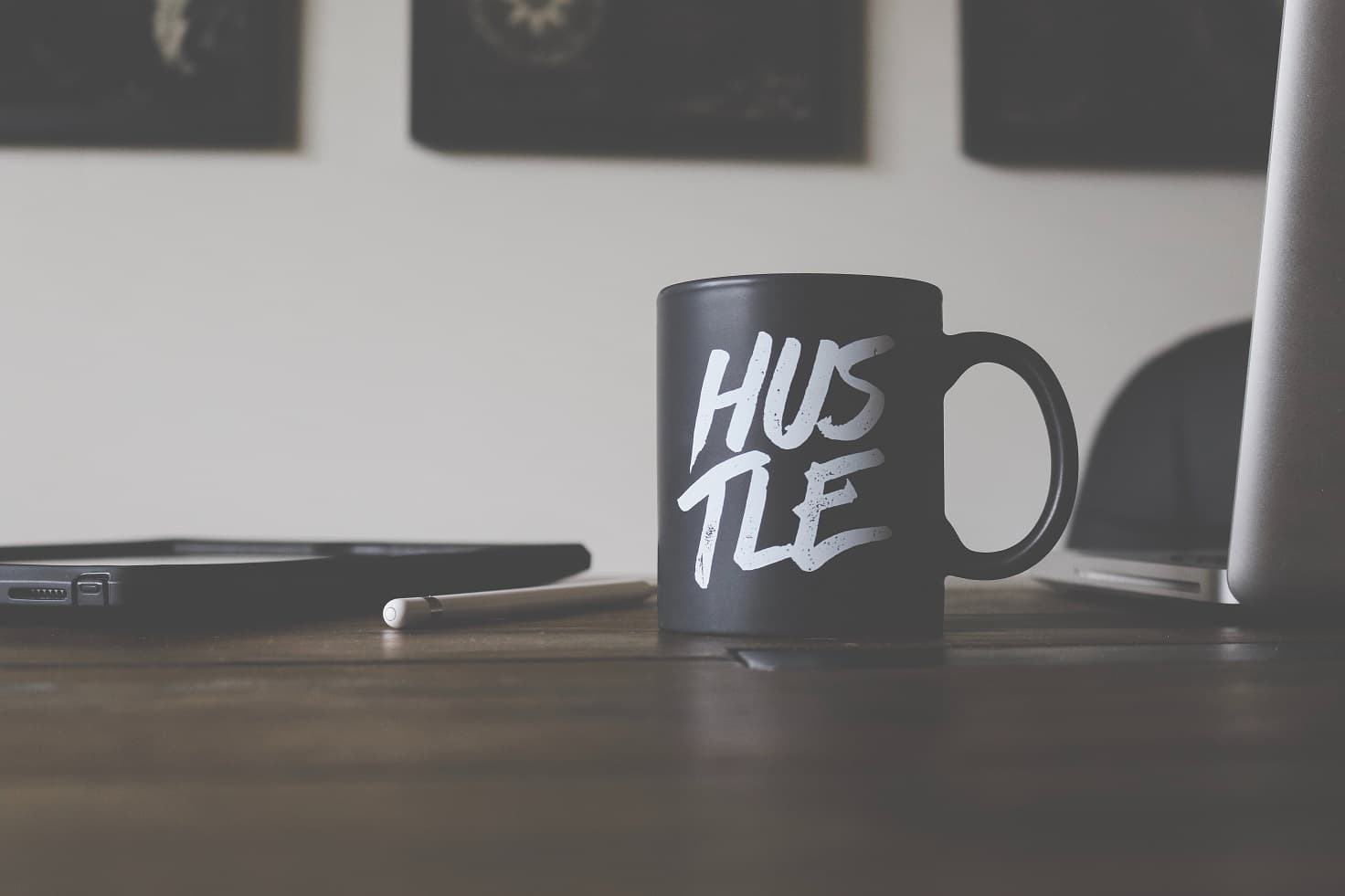
Photo by Garrhet Sampson on Unsplash
So you want to be a front-end developer? Awesome! Let me walk you through how I got started and what worked for me.
The HTML Adventure Begins
I used to think HTML was some kind of complicated programming language that only computer geniuses could understand. Boy, was I wrong! HTML turned out to be more like learning a new set of punctuation marks - weird-looking ones with angles and slashes, but punctuation nonetheless. It's really just a way of telling web browsers, "Hey, this part is a heading," or "This stuff belongs in a paragraph," or "Please, for the love of all things holy, make this text bold because it's important!" I spent about two weeks just playing around with different tags, building hideous websites about my pets that thankfully never saw the light of day. The <h1>
tag became my best friend - everything was important in those early days! And don't even get me started on my first attempts at tables - I somehow managed to create nested tables so complex they probably violated some laws of physics. But that's the beauty of HTML - you can mess up spectacularly and still learn something. I remember the websites I used to learn from had these interactive exercises where you could type code and see the results immediately. I'd spend hours tweaking and adjusting, feeling like a mad scientist every time something worked as intended. The best part? There are so many free resources out there. I bounced between W3Schools (which explained things in a way my non-technical brain could understand), MDN (which I initially found intimidating but grew to love for its depth), and countless YouTube tutorials where enthusiastic developers showed me tricks I hadn't even thought to look for. If you're just starting out, don't worry about perfection - just get your hands dirty with code and watch as plain text transforms into structured web content before your eyes.
CSS: The Art of Making Things Pretty (Eventually)
After fumbling my way through HTML, I thought, "How hard could CSS be? It's just making things pretty, right?" Ha! Little did I know I was stepping into a world where boxes don't always behave like boxes, where centering something vertically would become the bane of my existence, and where I'd spend three hours trying to figure out why a margin was pushing everything out of whack (spoiler: it was collapsing margins, a concept apparently designed to test the patience of budding developers). My first CSS attempts were... colorful, to say the least. I went through a phase where every website I built looked like it had been designed by someone with access to every crayon in the box and a mandate to use them all. Gradually though, I started to understand the logic behind the madness. I learned that CSS isn't just about colors and fonts, but about creating layouts that work across different devices and screen sizes. I discovered the joy (and occasional terror) of the box model, where padding, borders, and margins all played their part in the grand scheme of things. The biggest game-changer for me was when I finally understood how selectors work - suddenly I could target specific elements with surgical precision instead of applying styles with all the subtlety of a sledgehammer. I spent countless evenings on CSS-Tricks, marveling at the solutions to problems I hadn't even encountered yet. I pored over designs on Dribbble and Behance, trying to reverse-engineer how they achieved certain effects. And slowly, painfully, my designs started to look less like they belonged in the 1990s and more like something from the current decade. If you're diving into CSS, my advice is simple: be patient with yourself. This isn't something you master overnight. Build lots of small projects, try to recreate designs you admire, and don't be afraid to inspect the code of websites you love to see how they did it.
JavaScript: Where the Magic (and Confusion) Happens
I approached JavaScript with the confidence of someone who had no idea what they were getting into. "I've got HTML and CSS down," I thought. "How much harder could adding some interactivity be?" The answer, as it turned out, was "significantly harder." My first JavaScript program was supposed to be a simple calculator. After three days of work, it could add numbers... sometimes. Other times it would concatenate them (why does 2 + 2 equal 22?), and occasionally it would just throw errors that made absolutely no sense to me. I nearly gave up multiple times, convinced that programming just wasn't for me. But then something clicked. I realized I was thinking about JavaScript all wrong. It wasn't just HTML and CSS with some extra features - it was a proper programming language with its own logic, rules, and quirks. Once I adjusted my mindset and started from scratch with basic concepts like variables, functions, and control flow, things started to make more sense. The real breakthrough came when I built my first interactive website element - a simple dropdown menu that expanded when clicked. It was nothing fancy, but I had made something happen on the screen through code I'd written myself! That feeling of empowerment was addictive. From there, I tackled more complex challenges: form validation, API calls to fetch weather data, a small game where you had to catch falling objects. Each project taught me something new and reinforced what I'd learned before. I found that real-world projects, even small ones, taught me more than any tutorial could. I'd recommend starting with tiny, achievable goals - maybe a toggle button, or a form that checks if an email address is valid. Build your confidence with these small wins before moving on to bigger challenges. And don't just copy code from tutorials - type it out yourself, play with it, break it, fix it, and make it your own. That's how you really learn.
// My first JavaScript program that actually worked!
console.log('Hello, World!')
CSS Frameworks: The Day I Stopped Fighting the Grid
After months of wrestling with CSS to create layouts that didn't fall apart if you so much as looked at them wrong, I discovered CSS frameworks, and it was like someone had thrown me a lifeline in the stormy sea of web development. My first experience was with Bootstrap, and I still remember the shock of building a responsive navigation bar in minutes instead of days. It felt like cheating! I went a bit framework-crazy after that, jumping from Bootstrap to Foundation to Materialize, trying to figure out which one I liked best. Each had its own personality and quirks. Bootstrap was like that friend who's super reliable but sometimes a bit boring - everything looked like a Bootstrap site unless you really customized it. Materialize brought Google's Material Design principles to life in a way that made my apps look modern and professional without much effort on my part. But the framework that really changed how I thought about CSS was Tailwind. At first, I hated it. All those utility classes cluttering up my HTML felt wrong after I'd spent so long learning to separate content and styling. But after pushing through that initial resistance, I realized how incredibly fast I could build and iterate with it. No more switching between files, no more thinking up class names, just rapid development right in my HTML. I think the key with frameworks is to understand what they're doing under the hood rather than treating them as magic. I made sure to learn the grid system concepts, not just the specific classes for a particular framework. This meant I could switch between frameworks more easily or even drop back to vanilla CSS when needed. If you're just starting out, I'd suggest picking one framework and getting really comfortable with it before trying others. And don't feel like you need to use a framework for everything - sometimes vanilla CSS is still the best tool for the job, especially for smaller projects or when you need something very specific.
CSS Preprocessors: The Day I Said Goodbye to Repetitive Code
I still remember the day a senior developer looked over my shoulder, saw me copying and pasting the same color hex code for the fifth time, and said, "You know you could just use a variable for that, right?" I had no idea what he was talking about until he introduced me to Sass. It was one of those moments where you realize there's a whole world of tools designed to solve problems you didn't even know were problems. My first reaction to Sass was skepticism - did I really need to learn another thing just to write CSS? But after a day of playing with variables, nesting, and mixins, I was sold. Suddenly all the repetitive parts of my CSS were gone, replaced with elegant, maintainable code. I could define a color palette once and reference it throughout my stylesheet. I could nest selectors to match my HTML structure, making the relationship between elements much clearer. And mixins let me define reusable chunks of CSS that I could drop in wherever needed, which was a game-changer for cross-browser compatibility issues. The transition wasn't without hiccups, of course. Setting up a preprocessing workflow was confusing at first - all those build tools and config files were intimidating. I started with simple online converters before gradually setting up a proper development environment with gulp (and later npm scripts). I spent a weekend converting an existing CSS project to Sass, and despite the initial time investment, maintenance and updates became so much faster afterward. If you're on the fence about preprocessors, I'd say start small. You don't need to use every feature right away. Begin with variables for colors and font sizes, then gradually incorporate nesting and mixins as you get comfortable. The beauty of preprocessors like Sass and Less is that valid CSS is also valid Sass/Less, so you can adopt features incrementally rather than all at once.
JavaScript Frameworks: When Vanilla JS Just Isn't Enough
I resisted JavaScript frameworks for longer than I care to admit. "I can build this with vanilla JS," I'd insist, spending hours reinventing wheels that had already been perfectly crafted by others. My first real project with a framework was a React to-do app - yeah, I know, the "hello world" of framework projects, but it was a revelation to me. The way React handled state and updated only what needed to be updated was mind-blowing compared to my previous method of manually updating the DOM. I quickly became a React enthusiast, building everything from simple apps to complex dashboards with it. But then I got curious about other frameworks. Vue caught my attention with its gentle learning curve and clear documentation. I rebuilt that same to-do app in Vue and was surprised at how intuitive it felt. Then came Svelte, which compiled away to vanilla JavaScript and had a completely different approach. Each framework had its strengths, and I found myself reaching for different tools depending on the project requirements. React for complex applications with lots of state management, Vue for projects where I was working with designers who needed to understand the code, Svelte for performance-critical applications. The most important lesson I learned wasn't which framework was "best" (they all have their place), but rather the core concepts that underpin all of them: component-based architecture, state management, reactive updates, and the virtual DOM. Understanding these concepts meant I could pick up new frameworks more quickly and make informed decisions about which to use for a particular project. If you're just starting with frameworks, don't feel like you need to learn them all at once. Pick one that interests you or is popular in your area or industry, and really get to know it before branching out. And remember, the goal isn't to use a framework because it's cool or popular, but because it solves a specific problem you're facing better than the alternatives.
The Practice Makes Perfect (Or At Least Better) Phase
There's a world of difference between understanding a concept and being able to apply it effectively. I learned this the hard way when I thought I had CSS Grid all figured out after a few tutorials, only to struggle for hours implementing a seemingly simple layout. That's when I realized that reading and watching could only take me so far - I needed to build, break, fix, and build again to truly internalize these skills. My practice approach became more structured over time. Instead of jumping into complex projects right away, I started breaking things down into smaller, focused exercises. If I was learning flexbox, I'd spend an evening just creating different layouts with it - navigation bars, card layouts, centering content both horizontally and vertically. I'd set myself challenges: "Recreate this website header without looking at their code," or "Build this complex layout that adjusts gracefully from mobile to desktop." I found that participating in coding challenges like Frontend Mentor or JavaScript30 gave me practical problems to solve and exposed me to techniques I might not have discovered on my own. Contributing to open-source projects was initially terrifying (what if my code wasn't good enough?), but it turned out to be one of the most valuable learning experiences. Having my pull requests reviewed by experienced developers showed me best practices and alternative approaches I wouldn't have considered. The most important thing I learned about practice is that consistency trumps intensity. Twenty minutes of coding every day is far more effective than a single eight-hour marathon on the weekend. And don't underestimate the value of revisiting basics periodically - I still occasionally rebuild simple projects from scratch to reinforce fundamentals and see how my approach has evolved. If you're just starting your practice journey, find small projects that excite you. Personal interest goes a long way toward maintaining motivation when you hit inevitable roadblocks. Build things you'd actually use, or solve problems you encounter in your daily life. And don't forget to step back occasionally and reflect on how far you've come - progress in programming can sometimes feel slow day-to-day, but tends to be impressive when viewed over months or years.
Version Control: The Day I Stopped Fearing Change
My introduction to version control was both humbling and liberating. I'd been working on a personal project for weeks, making changes directly to the files, when disaster struck - I made a series of "improvements" that completely broke everything, and I had no way to go back to the working version. After a day of trying to fix it, I had to start over from scratch. Never again, I promised myself. A developer friend took pity on me and spent an afternoon introducing me to Git. At first, it seemed needlessly complex - all these commands and concepts like repositories, branches, commits, and the mysterious "staging area" that seemed like an extra step I didn't need. But the first time I confidently made a significant change to my code, knowing I could easily revert if things went south, I was converted. Version control gave me the freedom to experiment without fear. I could try bold new approaches, and if they didn't work out, no problem - just revert to the previous commit. I could work on multiple features simultaneously using branches, without them interfering with each other. And when I started collaborating with other developers, Git became even more valuable. We could work on the same codebase without overwriting each other's changes, and the commit history served as a record of why certain decisions were made. Learning Git wasn't a straight path. I made plenty of mistakes - committing files I shouldn't have, creating merge conflicts that seemed impossible to resolve, accidentally deleting branches with work I hadn't pushed. But each mistake taught me something new about how Git worked under the hood. If you're new to version control, my advice is to start using it even for personal projects. Create a GitHub account and push your code there - not only does it serve as a backup, but it also starts building your portfolio for potential employers. Don't worry about mastering every Git command at once; focus on the basic workflow (clone, add, commit, push, pull) and expand your knowledge as needed. And remember, even experienced developers Google Git commands regularly - it's not about memorization but understanding the concepts.
Web Design Principles: When I Realized Development Isn't Just About Code
I used to think that as a developer, my job was just to implement the designs that designers created. Why would I need to understand design principles? This mindset changed when I started working on personal projects where I had to wear both the designer and developer hats. My first attempts were... not great. Functional? Yes. Aesthetically pleasing? Not even close. I realized that to create truly compelling websites, I needed at least a basic understanding of design principles. Color theory was my first deep dive. I learned about color harmonies, the psychology of different colors, and how to create palettes that worked together instead of fighting for attention. Tools like Coolors and Adobe Color became essential parts of my workflow, helping me choose colors that complemented each other and served the purpose of the website. Typography came next. I discovered that good typography isn't just about choosing a nice font, but about hierarchy, readability, line length, line height, and dozens of other considerations that most people never consciously notice but that dramatically affect the user experience. I experimented with different font pairings, learning which combinations worked well together and which clashed. Layout design was perhaps the most challenging aspect to master. I had to train my eye to recognize when things were unbalanced or when there was too much (or too little) whitespace. I learned about the rule of thirds, the golden ratio, and how to create visual hierarchies that guided users through content in the intended order. What surprised me most was how understanding these principles made me a better developer. I could anticipate design challenges before they arose, suggest alternatives when technical limitations prevented implementing a design exactly as specified, and communicate more effectively with designers. If you're primarily focused on development, I'd still encourage you to spend some time learning basic design principles. You don't need to become an expert, but understanding the "why" behind design decisions will make you more versatile and valuable. Study websites you admire, not just for their code but for their design choices. Ask yourself why certain elements are positioned or styled the way they are. And don't be afraid to experiment with your own designs, even if they don't turn out perfectly at first.
Are you still with me on this front-end development journey? Great! Let's keep going through some more essential skills that have saved my bacon countless times.
Debugging: The Sherlock Holmes Phase of Development
I used to have a love-hate relationship with bugs in my code. On one hand, they were frustrating obstacles keeping me from finishing my work. On the other hand, successfully hunting down and fixing a particularly elusive bug gave me a satisfaction unlike anything else. My approach to debugging in the early days was... chaotic, to put it kindly. I'd make random changes, hoping something would magically fix the issue. When that inevitably failed, I'd resort to littering my code with console.log statements, trying to track down where things went wrong. It was like looking for a needle in a haystack while blindfolded. Everything changed when I properly learned to use browser developer tools. Chrome DevTools became my best friend – the Elements panel for inspecting and modifying the DOM, the Network tab for tracking requests and responses, and most importantly, the Sources panel for setting breakpoints and stepping through my JavaScript code line by line. I remember the first time I used the debugger statement in my JavaScript – it was like suddenly having X-ray vision into my code's execution. Instead of guessing what was happening, I could watch variables change in real-time and pinpoint exactly where errors occurred. Another game-changer was learning to read error messages properly. Initially, I'd see a wall of red text in the console and panic. But I gradually realized that error messages are actually incredibly helpful if you take the time to understand them. They tell you what went wrong, where it happened, and often give clues about how to fix it. The call stack, which I'd ignored for months, turned out to be a roadmap leading directly to the source of problems. These days, my debugging process is much more methodical. When something breaks, I first look for error messages in the console. If there aren't any obvious errors, I formulate hypotheses about what might be going wrong and test them systematically. I use breakpoints strategically rather than randomly, and I've learned to utilize advanced features like conditional breakpoints and watch expressions. If you're struggling with debugging, remember that it's a skill that improves with practice. Embrace bugs as learning opportunities rather than frustrations. Get comfortable with your browser's developer tools – invest time in learning keyboard shortcuts and less obvious features. And perhaps most importantly, develop a methodical approach rather than making random changes and hoping for the best.
Staying Current: Riding the Wave of Constant Change
One of the most intimidating aspects of front-end development when I was starting out was the pace of change. It seemed like every week there was a new framework, library, or tool that everyone was suddenly talking about. How could anyone possibly keep up? I tried to learn everything at first, jumping from tutorial to tutorial and never really mastering anything. It was exhausting and unproductive. Eventually, I realized that chasing every new shiny technology was a fool's errand. Instead, I needed to focus on understanding core principles and concepts that wouldn't change as quickly, while selectively adopting new tools that offered significant benefits for my specific work. I developed a system to stay informed without being overwhelmed. I subscribed to a few high-quality newsletters like JavaScript Weekly and Frontend Focus that curated the most important developments. I followed a handful of influential developers on Twitter who shared insights about emerging trends. And I set aside a few hours each week for learning and experimentation – sometimes exploring new technologies, sometimes deepening my understanding of tools I already used. Community involvement became crucial for my continued growth. I joined local meetup groups and online communities where developers shared knowledge and experiences. These connections were invaluable not just for learning about new technologies, but for understanding which ones were actually being adopted in production environments versus those that were just generating buzz. Perhaps most importantly, I learned to evaluate new technologies critically rather than adopting them reflexively. For each new tool or framework, I'd ask questions like: Does this solve a real problem I'm facing? Is it mature enough for production use? Does it have community support and documentation? Will it still be around in a year or two? This approach has saved me countless hours that might have been spent learning technologies that didn't stand the test of time. If you're feeling overwhelmed by the pace of change, remember that it's impossible to learn everything, and that's okay. Focus on mastering fundamentals first, as they'll serve you well regardless of which specific technologies are in favor. Be strategic about what you learn, prioritizing technologies that are relevant to your interests and career goals. And don't forget that learning how to learn is ultimately more valuable than knowledge of any particular framework or library.
Responsive Design: When I Realized Mobile Wasn't Just an Afterthought
I still cringe when I think about my early websites. They looked great on my desktop monitor, but open them on a phone and... disaster. Text running off the screen, elements overlapping, tiny unclickable buttons – it was a mess. This was before responsive design had become the standard approach, and my solution was to create separate "mobile versions" of websites, essentially maintaining two codebases. Not surprisingly, this was a maintenance nightmare. My responsive design journey began in earnest when I discovered media queries. Suddenly I could adjust layouts, font sizes, and spacing based on the device's screen size, all within a single codebase. My first attempts were basic – I'd design for desktop first, then add media queries to fix things for smaller screens. This often led to bloated CSS and awkward compromises. The real breakthrough came when I adopted a mobile-first approach. Starting with the mobile layout forced me to focus on content priorities and essential functionality, then progressively enhance the experience for larger screens. This resulted in leaner code and better experiences across all devices. Frameworks like Bootstrap helped accelerate my learning by providing ready-made responsive components and grid systems. I could see how experienced developers structured responsive layouts and gradually incorporated those patterns into my own custom code. Responsive images were another challenge altogether. I learned about the srcset attribute and picture element, which allowed me to serve appropriately sized images based on screen size and resolution, significantly improving performance on mobile devices. The most valuable lesson I learned about responsive design is that it's not just about making things fit on different screens – it's about creating appropriate experiences for different contexts. A user on a phone might have different needs and priorities than a user on a desktop, and good responsive design accounts for these differences. If you're working on improving your responsive design skills, I'd recommend starting with a simple existing project and retrofitting it to be responsive. This practical experience will teach you more than any tutorial. Use browser developer tools to simulate different screen sizes, and test on real devices whenever possible. Remember that responsive design is not a feature to be added at the end of a project, but an approach that should inform your development process from the beginning.
Building a Portfolio: From Zero to Employable
When I decided to pursue front-end development professionally, I faced a chicken-and-egg problem: I needed experience to get a job, but how could I get experience without a job? The answer, of course, was building a portfolio of personal projects. My first portfolio was embarrassingly basic – a simple HTML page with links to a few small projects. But it was a start, and gave me something to show potential employers beyond just claiming "I know HTML, CSS, and JavaScript." As my skills improved, so did my portfolio. I rebuilt it from scratch several times, each iteration showcasing new techniques I'd learned. The portfolio itself became evidence of my abilities, not just the projects it contained. I discovered that quality trumped quantity when it came to portfolio projects. Three polished, well-executed projects impressed interviewers more than a dozen half-finished experiments. I focused on creating projects that demonstrated different skills – one highlighting UI design abilities, another showcasing API integration, a third demonstrating complex state management with React. Documentation became an unexpected differentiator. For each project, I created a detailed README explaining the project's purpose, the technologies used, challenges overcome, and lessons learned. This gave interviewers insight into my thinking process and problem-solving approach, not just my coding abilities. I also made sure to include the code on GitHub (with clean, commented code) and deploy working versions so people could interact with them easily. The feedback loop was crucial for improvement. I shared my portfolio with more experienced developers and incorporated their suggestions. I paid attention to questions interviewers asked about my projects and enhanced my documentation to address common queries. Building case studies around my projects was particularly effective. Instead of just showing what I built, I explained the problem I was solving, my approach to the solution, alternative approaches I considered, and reflections on what I'd do differently next time. This demonstrated critical thinking and professional maturity that set me apart from other candidates with similar technical skills. If you're building your portfolio, remember that it's not just a collection of projects but a representation of you as a developer. Choose projects that you're genuinely interested in so your passion shows through. Don't be afraid to include non-traditional projects if they demonstrate valuable skills. And keep iterating – your portfolio should evolve as your skills grow.
Now let's tackle some advanced topics that can really set you apart as a front-end developer.
Accessibility: The Day I Realized Not Everyone Experiences the Web the Same Way
I'd been developing websites for over a year before accessibility even crossed my mind. I was designing for people like me – young, tech-savvy, with no visual or motor impairments. My wake-up call came during an internship when I watched a user with visual impairments try to navigate a website I'd helped build. Their screen reader jumped erratically around the page, skipping crucial information and getting stuck in navigation loops. Features I thought were intuitive were completely unusable. I was mortified, but also motivated to do better. My accessibility education began with understanding the diverse ways people interact with websites. Some users rely entirely on keyboards or specialized input devices, never using a mouse. Others use screen readers that translate visual information into speech or braille. Some need high contrast or larger text to read comfortably. Many have temporary limitations – imagine trying to use a website one-handed while holding a baby, or in bright sunlight that makes low-contrast text invisible. I started with simple improvements: adding proper alt text to images, ensuring keyboard navigation worked logically, using semantic HTML elements instead of generic divs for everything, and checking that color combinations had sufficient contrast. These basic changes made a significant difference without requiring major redesigns. The Web Content Accessibility Guidelines (WCAG) became my reference point for more comprehensive improvements. I learned about ARIA attributes that could provide additional context to assistive technologies, and about how to structure forms, tables, and custom components to be accessible. Testing became an essential part of my process. I learned to use my keyboard exclusively to navigate my sites, to test with screen readers like NVDA and VoiceOver, and to use browser extensions that simulated various visual impairments. Each method revealed different issues that might otherwise have gone unnoticed. What surprised me most was how many accessibility improvements benefited all users, not just those with disabilities. Clearer navigation, better form labels, and more consistent interactions made sites better for everyone. And many accessibility features turned out to be great for SEO as well – properly structured headings and descriptive link text helped search engines understand content just as they helped screen readers. If you're looking to improve the accessibility of your projects, start with an audit of an existing site using a tool like WAVE or Lighthouse. The results might be overwhelming at first, but focus on fixing one category of issues at a time. Remember that perfect accessibility isn't achieved overnight – it's an ongoing process of improvement and learning.
Performance Optimization: When Every Millisecond Counts
My first real encounter with performance optimization came when a client complained that their newly launched website was "sluggish." I was confused – it loaded fine on my high-end development machine with its fiber internet connection. But when I tested on a mid-range smartphone over a 3G connection, the truth became painfully clear. The site took over 20 seconds to become interactive, an eternity in web time. Users would have abandoned it long before seeing the content. That experience taught me an important lesson: we don't develop for ourselves or our machines, but for a diverse audience with varying devices and connection speeds. My performance journey started with the basics: compressing images, minifying CSS and JavaScript, and enabling browser caching. These simple optimizations cut loading time in half, but I knew there was more to be done. I delved deeper into performance metrics, learning the difference between metrics like First Contentful Paint, Time to Interactive, and Cumulative Layout Shift. Each captured a different aspect of the user experience, and optimizing for all of them required different strategies. JavaScript optimization became a major focus. I learned to use tools like Webpack to bundle and split my code, loading only what was needed for the initial render and lazy-loading the rest. I discovered that some of my animation libraries were bloating my bundle size, and found lighter alternatives or custom solutions that achieved the same effect with a fraction of the code. I became obsessed with reducing HTTP requests, combining files where possible and using CSS sprites for interface elements. I implemented resource hints like preconnect and preload to establish early connections to third-party domains and load critical resources sooner. The most challenging optimizations involved manipulating how and when resources loaded. I prioritized above-the-fold content, ensuring users saw something useful while the rest of the page loaded in the background. I deferred non-critical JavaScript and styles, and learned to optimize the critical rendering path so browsers could render the page more quickly. Testing became essential to my optimization process. I used tools like WebPageTest, Lighthouse, and Chrome's Performance panel to identify bottlenecks and verify improvements. I set up performance budgets for my projects, establishing maximum sizes for images, scripts, and stylesheets, and automatically flagging when these were exceeded. If you're new to performance optimization, start with the low-hanging fruit: optimize images, enable compression, and minify code. Then use testing tools to identify your specific bottlenecks rather than making random optimizations. Remember that performance isn't just about load times, but about creating a smooth, responsive experience throughout the user's interaction with your site.
Design Tools: Bridging the Designer-Developer Divide
For the longest time, I viewed design tools as the exclusive domain of designers. Whenever I received a Photoshop or Sketch file, I'd struggle to extract what I needed, often resorting to taking screenshots and measuring pixels with digital rulers – an inefficient and error-prone process. Everything changed when I decided to properly learn these tools myself. I started with Photoshop, learning how to navigate layers, understand blend modes, and export assets at different resolutions. Suddenly, design files weren't mysterious black boxes but resources I could confidently work with. My relationship with designers improved dramatically as we began speaking the same language. As design tools evolved, so did my skills. I learned Sketch, which was purpose-built for UI design rather than photo editing, and found it much more intuitive for web work. The ability to easily export CSS values and measure distances between elements streamlined my workflow considerably. Figma was a game-changer when it entered the scene. Being browser-based and collaborative, it eliminated the file-sharing dance that had previously slowed down projects. I could see designers working in real-time, ask questions directly within the design, and even make minor adjustments myself when needed. The developer handoff features in modern design tools proved invaluable. I could inspect elements to get exact colors, dimensions, and font specifications, copy generated CSS, and export assets in multiple formats with a few clicks. This dramatically reduced implementation errors and saved hours of back-and-forth communication. Understanding design tools also gave me more credibility when discussing technical constraints with designers. I could suggest alternatives that would be easier to implement while still maintaining the design intent, create prototypes to demonstrate interaction issues, and contribute more meaningfully to design reviews. If you're a developer looking to improve your design tool skills, start with the basics of whichever tool is used in your workplace or community. You don't need to master every feature – focus on the aspects most relevant to development handoff. Many design tools offer free trials or limited free plans, and there are countless tutorials available online. The time investment will pay dividends in smoother workflows and better designer-developer collaboration.
Working with APIs: From Data Consumer to API Expert
My first experience with APIs was simply copy-pasting code from documentation, crossing my fingers, and hoping it worked. I had little understanding of what was happening under the hood – it felt like magic when data suddenly appeared in my application.
The turning point came when I built a weather app that broke spectacularly in production. Debugging revealed I hadn't handled API errors properly, didn't understand rate limits, and my authentication tokens were exposed in client-side code. This painful experience forced me to truly understand how APIs work.
I started with the fundamentals – learning about HTTP methods, status codes, and request/response structures. I discovered tools like Postman that let me experiment with API calls outside my application code, which was invaluable for testing and understanding responses before implementing them.
// My early API calls were simplistic with no error handling
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => displayData(data)); // What if the API is down?
// Later I learned proper error handling and status checking
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error(`API error: ${response.status}`);
}
return response.json();
})
.then(data => displayData(data))
.catch(error => {
console.error('Fetch problem:', error);
showFallbackUI();
});
Authentication was my next challenge. I learned about API keys, OAuth flows, and JWT tokens, and the security implications of each. I moved sensitive credentials to environment variables and server-side code, protecting them from being exposed in the browser.
As I grew more confident, I moved beyond just consuming APIs to designing them. I learned RESTful principles and explored GraphQL as an alternative. Building my own backend APIs gave me deeper appreciation for what makes an API developer-friendly – good documentation, consistent error handling, and thoughtful endpoint design.
Caching strategies became crucial as my applications scaled. I implemented localStorage caching for frequently accessed, rarely changing data, and learned to balance freshness against performance. Managing loading states and providing feedback during API operations significantly improved perceived performance.
If you're beginning your API journey, start with well-documented public APIs like GitHub's or Weather APIs. Use tools like Postman or Insomnia to explore them before writing code. Focus on proper error handling from day one – users will forgive slow apps but not broken ones. And remember that understanding APIs isn't just about making successful requests; it's about creating robust applications that gracefully handle the unpredictable nature of external services.
Conclusion: The Never-Ending Learning Journey
Looking back on my front-end development journey, from struggling with basic HTML tags to architecting complex applications, I realize the most valuable skill I've developed isn't any specific technology – it's learning how to learn. The field evolves too rapidly for anyone to rest on their knowledge.
What worked for me was building a strong foundation in the fundamentals while remaining curious about emerging tools and techniques. I found balance between depth and breadth, mastering core technologies while experimenting with new ones. Most importantly, I learned to solve real problems rather than chasing trendy technologies.
If you're starting your front-end journey, remember that everyone struggles at first. The polished websites and applications you admire were built by developers who once knew less than you do now. Be patient with yourself, celebrate small victories, and keep building. With persistence and curiosity, you'll surprise yourself with how far you can go.
The beauty of front-end development is that it sits at the intersection of technical skill and human experience. Your code doesn't just run on machines – it creates experiences for real people. That human connection is what makes this field so challenging, but also so rewarding.
So keep learning, keep building, and keep pushing your boundaries. The path to mastery is a marathon, not a sprint, and the journey itself is where the real value lies.